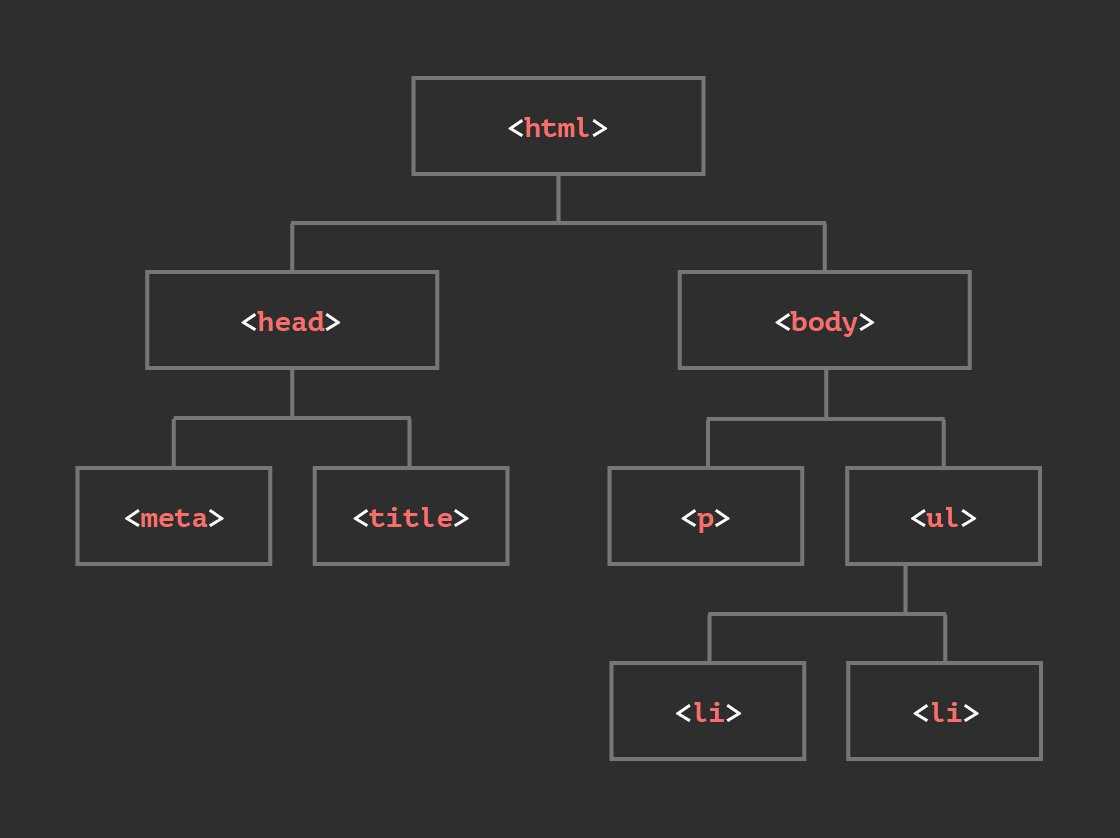
DOM Manipulation with Javascript
Informational
April 23, 2022
- Javascript
- DOM
- Document Object model
What's The DOM?
The Document Object Model (or DOM for short) is one of the most important parts with working on the web. The DOM represents the web page that's currently being worked on or visited, and allows the program to interact and change the document's structure.
The DOM is often thought about as a logical tree, breaking down the document structure and content so they can be consumed
and manipulated by Javascript. Although it's used in the web, it's important to note that the DOM is not part of
the Javascript library, but part of the Web Browser API. Other languages use DOM in their implementation, as well as
Javascript can run in environments outside of the browser. In order to start using the Document (or Window) API in Javascript,
all you have to do is include a <script>
tag in your HTML file.
To further enhance the range of possibilities with Javascript, using the DOM gives you access to manipulating HTML elements and inserting, deleteing or modifying them into your source code. The Element Interface has many different methods and properties, as well as the Document Interface. Paired together correctly can make for a powerful user experience.
DOM Manipulation
In order to start using the DOM in your javascript file, you simply reference the document
object in your file.
That will a wide range of options that include different properties and methods to start
editing your DOM.
The document
object has many different properties corresponding to the HTML file. Within the object, you get access to all
the nodes that is attached to whatever file structure is in place. Here are some of the most used properties that developers
use when working with the DOM.
document.documentElement
= The topmost element in the file. (<html>
)document.body
= DOM node that retrieves the body tag. (<body>
)document.head
= The head tag in an HTML file. (<head>
)
Another important thing to note is the children of the elements.
Searching the DOM
There are different ways to insert into the DOM. Below are some ways.
- document.getElementBy*
- document.querySelector*
It's important to note that it's no longer recommended to use getElementBy*
. querySelector*
simplifies the development process. For the blog's purpose, I'll still explain what the syntax for just those.
> querySelector()
Returns the HTML element that matches whatever selector parameter is passed in.
E.g:
const container = document.querySelector("#container");
// <div id="container">
const tag = document.querySelector(".tag");
// <div id="tag">
const loginInput = document.querySelector(
"div.user-panel.main input[name='login']"
);
{
/* <div>
<div class="user-panel main">
<input name="login"> // This would get returned
</div>
</div> */
}
> querySelectorAll()
Returns a list that matches whatever selector parameter is passed in.
E.g:
const matches = document.querySelectorAll("p");
// matches = [<p>, <p>, <p>]
const matches = document.querySelectorAll("div.highlighted > p");
{
/*
<div class="highlighted">
<p></p>
<p></p> // These p tags would get returned
<p></p>
</div>
*/
}
Inserting Into The DOM
One of the most important things to learn about DOM manipulation is learning how to insert into the DOM tree. When we write HTML, we have to statically type in our elements & those elements will forever be on that file unless altered by the developer. For example:
<body>
<div class="container">
<h1 class="greeting-header">Hello World!</h1>
</div>
</body>
This would display a very simple header tag with the message "Hello World!
" Now look at the code below.
<body>
<div class="container">
<h1 class="greeting-header">Hello World!</h1>
</div>
</body>
<script>
const container = document.querySelector(".container");
const p = document.createElement("p");
p.className = "paragraph";
p.innerText =
"This is something really cool being written into the DOM by JS.";
container.append(p);
</script>
Now what will be displayed is the same header tag we made before, but using javascript a new p tag with some text will be inserted.
A step by step process of what's going on is
- We insert a
<script>
tag so our file's Javascript has access to the DOM. - We create a reference to our body's element that has the
.container
class using the Document interface'squerySelector()
method - We create a new
<p>
element using Document interface'screateElement()
method. - We give our p reference a new class called "paragraph"
- Since the Element Interface in javascript is an object, using dot notation, we set the text inside of our empty
<p>
tag recently created to some filler text. - Lastly, we append (or attach) our
<p>
tag to our.container
class and we're done!
There are different type of methods we can use to insert something into the dom. The most common method would probably be
append()
but there are also other ways to insert.
- append() - insert into node, at the end
- prepend() - insert into node, at the beginning
- before() - insert right before node
- after() - insert right after node
Removing From The DOM
As you might imagine, you can also remove elements from the DOM. It's actually much simpler than insert since you can only remove an element that has already been written in the DOM.
const element = document.getElementById("demo");
element.remove();
DOM Properties
Using the idea that the DOM is just a javascript object, there is a wide range of manipulating that can be achieved. The Document interface lets you interact with many different attributes owned by the document, so this lets you use javascript to render anything you'd like. An easier way to think about it is attributes are written in HTML, while properties are written in the DOM's Object.
The browser "parses" (or reads") the HTML into DOM objects giving you access to all of the HTML elements and it's attributes. That way, you can locate something in Javascript by calling the DOM's properties. For example:
<body id="test-body">
<div>...</div>
</body>
const body = document.body.id; //test-body gets returned
As you can see above, javascript is using the document
object to retrieve the id of the body and setting it
a constant named "body." If we wanted to take it a step further, we can also change properties outside of the body tag using
the document
object. Another example below
document.title = "My Javascript DOM";
This would just simply change the title of the page. But now Javascript will let you dynamically change the title instead of just having a hardcoded title.
You can also dynamically modify attributes using the DOM. The document
object comes with different methods that
makes all attributes accessible.
elem.hasAttribute(name)
– checks for existence.elem.getAttribute(name)
– gets the value.elem.setAttribute(name, value)
– sets the value.elem.removeAttribute(name) –
removes the attribute.
If you wanted to check if a specific element has an attribute, this would be how to do so.
const el = document.querySelector("[custom-attribute]");
const value = el.getAttribute("[custom-attribute");
console.log(value); // set attribute in HTML will be returned
This is just a short blog to help explain the possibilities of what can be done using the DOM object, but there's so much more that is possible! If you want to learn more about what you can do, I recommend taking a look at MDN's pages regarding the DOM or Javascript.info's walkthrough on the DOM to learn more!